carg_t Struct Reference
Function argument. More...
#include <hexrays.hpp>
Inheritance diagram for carg_t:
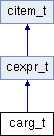
Public Member Functions | |
void | consume_cexpr (cexpr_t *e) |
DECLARE_COMPARISONS (carg_t) | |
![]() | |
bool | cpadone () const |
Pointer arithmetic correction done for this expression? More... | |
bool | is_odd_lvalue () const |
bool | is_fpop () const |
bool | is_cstr () const |
bool | is_type_partial () const |
bool | is_undef_val () const |
bool | is_jumpout () const |
bool | is_vftable () const |
void | set_cpadone () |
void | set_vftable () |
void | set_type_partial (bool val=true) |
cexpr_t (ctype_t cexpr_op, cexpr_t *_x, cexpr_t *_y=nullptr, cexpr_t *_z=nullptr) | |
cexpr_t (mba_t *mba, const lvar_t &v) | |
cexpr_t (const cexpr_t &r) | |
void | swap (cexpr_t &r) |
cexpr_t & | operator= (const cexpr_t &r) |
cexpr_t & | assign (const cexpr_t &r) |
DECLARE_COMPARISONS (cexpr_t) | |
void | replace_by (cexpr_t *r) |
Replace the expression. More... | |
void | cleanup () |
Cleanup the expression. More... | |
void | put_number (cfunc_t *func, uint64 value, int nbytes, type_sign_t sign=no_sign) |
Assign a number to the expression. More... | |
void | print1 (qstring *vout, const cfunc_t *func) const |
Print expression into one line. More... | |
void | calc_type (bool recursive) |
Calculate the type of the expression. More... | |
bool | equal_effect (const cexpr_t &r) const |
Compare two expressions. More... | |
bool | is_child_of (const citem_t *parent) const |
Verify if the specified item is our parent. More... | |
bool | contains_operator (ctype_t needed_op, int times=1) const |
Check if the expression contains the specified operator. More... | |
bool | contains_comma (int times=1) const |
Does the expression contain a comma operator? More... | |
bool | contains_insn (int times=1) const |
Does the expression contain an embedded statement operator? More... | |
bool | contains_insn_or_label () const |
Does the expression contain an embedded statement operator or a label? More... | |
bool | contains_comma_or_insn_or_label (int maxcommas=1) const |
Does the expression contain a comma operator or an embedded statement operator or a label? More... | |
bool | is_nice_expr () const |
Is nice expression? Nice expressions do not contain comma operators, embedded statements, or labels. More... | |
bool | is_nice_cond () const |
Is nice condition?. More... | |
bool | is_call_object_of (const citem_t *parent) const |
Is call object? More... | |
bool | is_call_arg_of (const citem_t *parent) const |
Is call argument? More... | |
type_sign_t | get_type_sign () const |
Get expression sign. More... | |
bool | is_type_unsigned () const |
Is expression unsigned? More... | |
bool | is_type_signed () const |
Is expression signed? More... | |
bit_bound_t | get_high_nbit_bound () const |
Get max number of bits that can really be used by the expression. More... | |
int | get_low_nbit_bound () const |
Get min number of bits that are certainly required to represent the expression. More... | |
bool | requires_lvalue (const cexpr_t *child) const |
Check if the expression requires an lvalue. More... | |
bool | has_side_effects () const |
Check if the expression has side effects. More... | |
bool | like_boolean () const |
Does the expression look like a boolean expression? In other words, its possible values are only 0 and 1. | |
bool | is_aliasable () const |
Check if the expression if aliasable. More... | |
uint64 | numval () const |
Get numeric value of the expression. More... | |
bool | is_const_value (uint64 _v) const |
Check if the expression is a number with the specified value. More... | |
bool | is_negative_const () const |
Check if the expression is a negative number. More... | |
bool | is_non_negative_const () const |
Check if the expression is a non-negative number. More... | |
bool | is_non_zero_const () const |
Check if the expression is a non-zero number. More... | |
bool | is_zero_const () const |
Check if the expression is a zero. More... | |
bool | is_value_used (const citem_t *parent) const |
Does the PARENT need the expression value. | |
bool | get_const_value (uint64 *out) const |
Get expression value. More... | |
bool | maybe_ptr () const |
May the expression be a pointer? More... | |
cexpr_t * | get_ptr_or_array () |
Find pointer or array child. More... | |
const cexpr_t * | find_op (ctype_t _op) const |
Find the child with the specified operator. More... | |
cexpr_t * | find_op (ctype_t _op) |
const cexpr_t * | find_num_op () const |
Find the operand with a numeric value. More... | |
cexpr_t * | find_num_op () |
const cexpr_t * | find_ptr_or_array (bool remove_eqsize_casts) const |
Find the pointer operand. More... | |
const cexpr_t * | theother (const cexpr_t *what) const |
Get the other operand. More... | |
cexpr_t * | theother (const cexpr_t *what) |
bool | get_1num_op (cexpr_t **o1, cexpr_t **o2) |
Get pointers to operands. More... | |
bool | get_1num_op (const cexpr_t **o1, const cexpr_t **o2) const |
const char * | dstr () const |
![]() | |
citem_t (ctype_t o=cot_empty) | |
void | swap (citem_t &r) |
Swap two citem_t. More... | |
bool | is_expr () const |
Is an expression? More... | |
bool | contains_expr (const cexpr_t *e) const |
Does the item contain an expression? More... | |
bool | contains_label () const |
Does the item contain a label? More... | |
const citem_t * | find_parent_of (const citem_t *sitem) const |
Find parent of the specified item. More... | |
citem_t * | find_parent_of (const citem_t *item) |
citem_t * | find_closest_addr (ea_t _ea) |
void | print1 (qstring *vout, const cfunc_t *func) const |
Print item into one line. More... | |
Public Attributes | |
bool | is_vararg = false |
is a vararg (matches ...) More... | |
tinfo_t | formal_type |
formal parameter type (if known) More... | |
![]() | |
union { | |
cnumber_t * n | |
used for cot_num More... | |
fnumber_t * fpc | |
used for cot_fnum More... | |
struct { | |
union { | |
var_ref_t v | |
used for cot_var More... | |
ea_t obj_ea | |
used for cot_obj More... | |
} | |
int refwidth | |
how many bytes are accessed? (-1: none) More... | |
} | |
struct { | |
cexpr_t * x | |
the first operand of the expression More... | |
union { | |
cexpr_t * y | |
the second operand of the expression More... | |
carglist_t * a | |
argument list (used for cot_call) More... | |
uint32 m | |
member offset (used for cot_memptr, cot_memref) for unions, the member number More... | |
} | |
union { | |
cexpr_t * z | |
the third operand of the expression More... | |
int ptrsize | |
memory access size (used for cot_ptr, cot_memptr) More... | |
} | |
} | |
cinsn_t * insn | |
an embedded statement, they are prohibited at the final maturity stage (CMAT_FINAL) More... | |
char * helper | |
helper name (used for cot_helper) More... | |
char * string | |
utf8 string constant, user representation (used for cot_str) More... | |
}; | |
tinfo_t | type |
expression type. must be carefully maintained More... | |
uint32 | exflags = 0 |
Expression attributes More... | |
![]() | |
ea_t | ea = BADADDR |
address that corresponds to the item. may be BADADDR More... | |
ctype_t | op = cot_empty |
item type More... | |
int | label_num = -1 |
label number. More... | |
int | index = -1 |
an index in cfunc_t::treeitems. More... | |
Detailed Description
Member Function Documentation
◆ consume_cexpr()
void carg_t::consume_cexpr | ( | cexpr_t * | e | ) |
Definition at line 6580 of file hexrays.hpp.
◆ DECLARE_COMPARISONS()
carg_t::DECLARE_COMPARISONS | ( | carg_t | ) |
Definition at line 6585 of file hexrays.hpp.
Member Data Documentation
◆ formal_type
tinfo_t carg_t::formal_type |
formal parameter type (if known)
- Examples
- hexrays_sample2.cpp.
Definition at line 6579 of file hexrays.hpp.
◆ is_vararg
bool carg_t::is_vararg = false |
is a vararg (matches ...)
Definition at line 6578 of file hexrays.hpp.